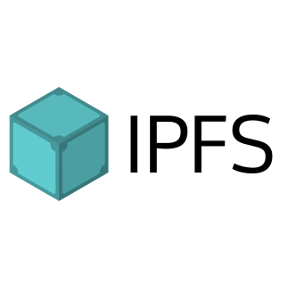
IPFS
A peer-to-peer hypermedia protocol designed to make the web faster, safer, and more open.
Use-Case
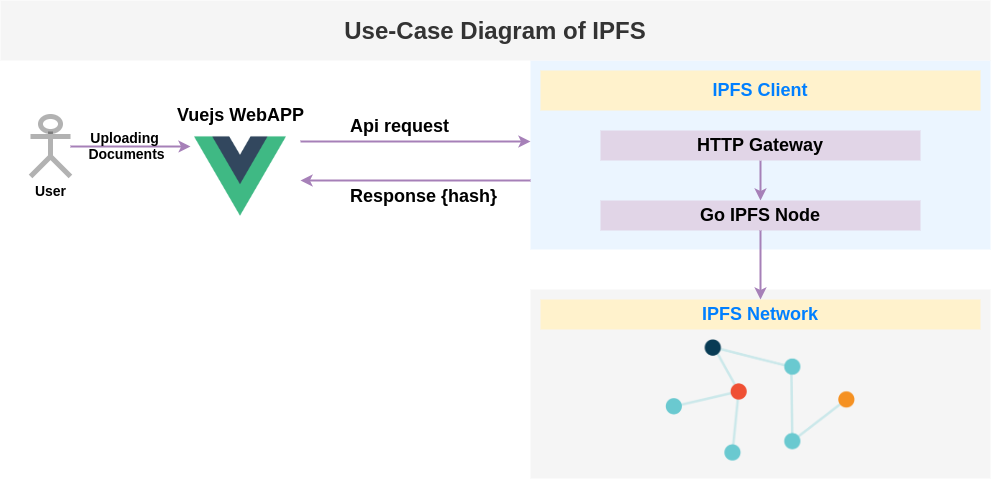
Step Flow Diagram
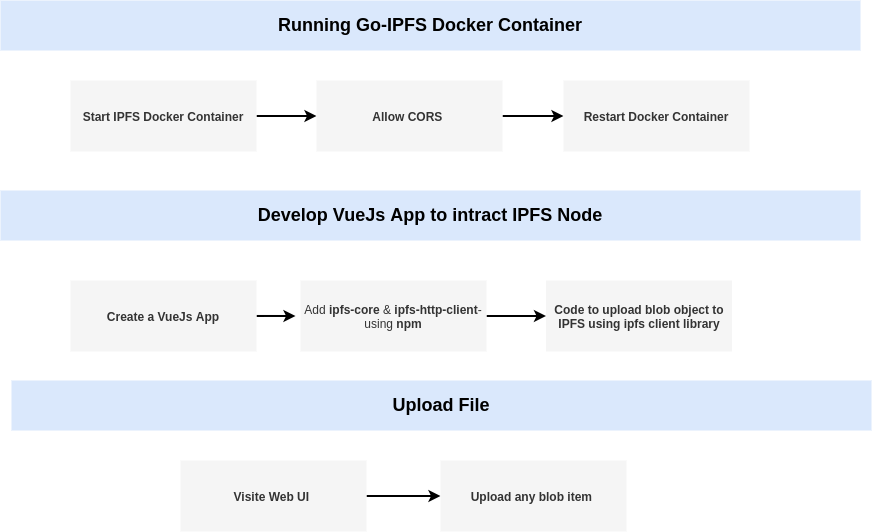
Prerequisites
Docker
Npm
Node
Vue Cli
Installation
Create IPFS docker container
docker run -d \ --name ipfs_host \ -v ipfs_staging:/export \ -v ipfs_data:/data/ipfs \ -p 4001:4001 \ -p 8080:8080 \ -p 5001:5001 \ ipfs/go-ipfs:latest
Bash into docker container
docker exec -it ipfs_host sh
Allow CORS
ipfs config Addresses.Gateway /ip4/0.0.0.0/tcp/8080 ipfs config Addresses.API /ip4/0.0.0.0/tcp/5001 ipfs config --json API.HTTPHeaders.Access-Control-Allow-Origin "[\"*\"]" ipfs config --json API.HTTPHeaders.Access-Control-Allow-Credentials "[\"true\"]"
Exit
andRestart
docker containerdocker restart ipfs_host
Getting Started
Create a new Vue app
vue create ipfs-demo
Add following packages to the project
Vuetify
vue add vuetify
vue-router
vue add router
ipfs-core and ipfs-http-client
npm install ipfs-core ipfs-http-client
Clean up the project
Delete components folder
Delete views folder contents
Clean App.vue file Contents
Clear router/index.js file Contents
Insert Below code inside
App.vue
<template> <v-app> <v-app-bar app color="primary" dark >IPFS Demo</v-app-bar> <v-main app> <router-view /> </v-main> </v-app> </template>
Insert below code snippet in
views/ipfsFileUpload.vue
<template>
<v-container>
<v-file-input
v-model="chosenFile"
dense
outlined
label="File input"
></v-file-input>
<v-btn @click="fileUpload">Upload</v-btn>
<v-dialog v-model="loaderDialog" hide-overlay persistent width="300">
<v-card color="primary" dark>
<v-card-text>
Please stand by
<v-progress-linear
:value="progress"
indeterminate
color="white"
class="mb-0"
></v-progress-linear>
</v-card-text>
</v-card>
</v-dialog>
<v-dialog v-model="resultDialog" hide-overlay persistent width="500">
<v-card color="primary" class="text-center" dark>
{{ this.message }}
<br />
<v-btn @click="resultDialog = !resultDialog">Ok</v-btn>
</v-card>
</v-dialog>
</v-container>
</template>
<script>
const ipfsClient = require("ipfs-http-client");
const ipfs = ipfsClient("http://localhost:5001");
export default {
name: "ipfsFileUpload",
data() {
return {
chosenFile: undefined,
progress: 0,
loaderDialog: false,
resultDialog: false,
message: "",
};
},
methods: {
async fileUpload() {
this.progress = 0;
let file = this.chosenFile;
let fileSize = file.size;
try {
this.loaderDialog = true;
const result = await ipfs.add(file, {
progress: (prog) => {
this.progress = (prog * 100) / fileSize;
console.log(`received: ${prog}`);
},
});
let url = `https://ipfs.io/ipfs/${result.path}`;
console.log(url);
this.message = `File Uploaded Successfully \n URL: ${url}`;
this.loaderDialog = false;
this.resultDialog = true;
} catch (err) {
this.message = "Please Upload File";
console.error(err);
}
},
},
};
</script>
Insert below code snippet in
router/index.js
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) const routes = [ { path: '/', redirect : '/ipfs', }, { path: '/ipfs', name: 'ipfsFileUpload', component: () => import('../views/ipfsFileUpload.vue') } ] const router = new VueRouter({ mode: 'history', base: process.env.BASE_URL, routes }) export default router
Start Vue.js app
npm run serve
View IPFS demo webapp at:
http://localhost:8081/
.Upload file and access via generated URL